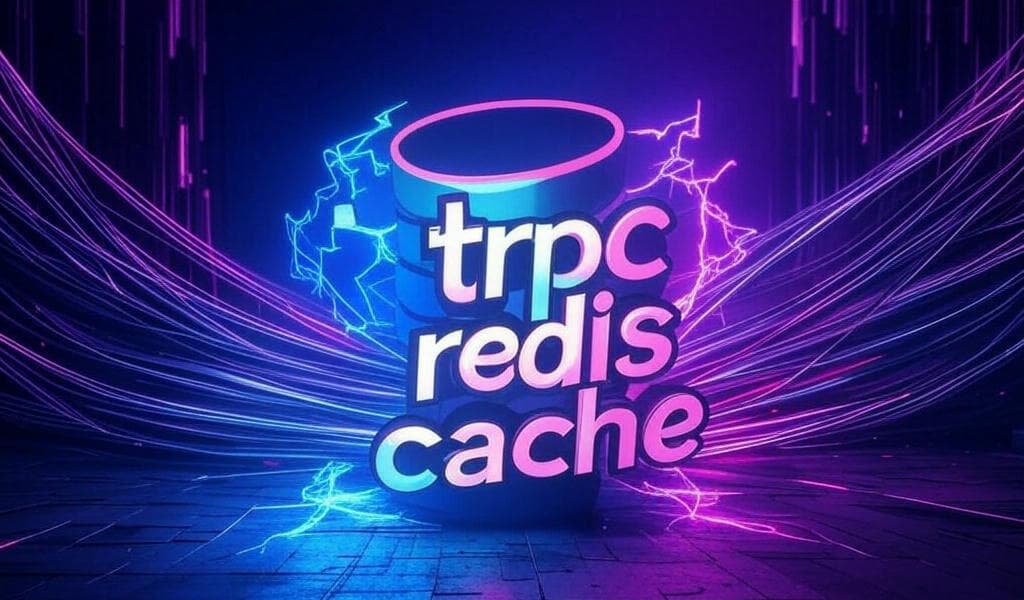
Introducing trpc-redis-cache: Simple and Powerful Caching for Your tRPC Procedures
If you’ve ever built a web application using tRPC, you’ve likely encountered the need to cache expensive operations. Whether it’s complex database queries, third-party API calls, or heavy computational tasks, caching is a must-have for efficient and scalable applications. But caching can quickly get complicated—especially when your app grows, and caching needs become more nuanced. This is precisely where our new package, trpc-redis-cache, comes into play.
🚧 The Problem with Manual Caching
Implementing caching manually is often repetitive, error-prone, and can lead to inconsistencies. Manually managing cache keys, invalidations, and handling user-specific caches becomes messy fast. Even a minor mistake can result in stale data, negatively affecting your user experience.
Developers deserve a simple, elegant solution that fits neatly into their existing tRPC ecosystem—without sacrificing flexibility or power.
💡 A Humble Solution: Meet trpc-redis-cache
We built trpc-redis-cache to provide a straightforward yet powerful middleware solution for tRPC procedures. With just a few lines of code, you can integrate Redis caching seamlessly into your tRPC setup. It’s designed to be intuitive, customizable, and reliable.
🔥 Features at a glance:
- Easy Setup: Plug-and-play middleware integration with minimal configuration.
- Flexible Backend: Supports standard Redis instances and serverless solutions like Upstash Redis.
- User-specific and Global Caching: Effortlessly manage caches at a global level or specific to individual users.
- Customizable Keys & TTL: Precisely control cache expiration times and key generation logic.
- Built-in Logging & Debugging: Easily track cache hits, misses, and performance.
- Simple Cache Invalidation: Quickly invalidate cache entries when your underlying data changes.
🚀 Getting Started is Effortless
Installation couldn’t be simpler:
pnpm add trpc-redis-cache
# or
npm install trpc-redis-cache
# or
yarn add trpc-redis-cache
Then add it to your tRPC procedure:
import { createCacheMiddleware } from 'trpc-redis-cache';
// Create cache middleware with default options
const cacheMiddleware = createCacheMiddleware();
const appRouter = createTRPCRouter({
getUser: protectedProcedure
.input(z.object({ id: z.string() }))
.use(cacheMiddleware)
.query(async ({ input }) => {
// This operation will now be cached automatically!
return await getUserById(input.id);
}),
});
⚙️ Advanced Customization: A Flexible Approach
For more advanced use cases, trpc-redis-cache empowers you with granular control:
- Permanent Global Cache:
const globalCacheMiddleware = createCacheMiddleware({
ttl: undefined, // never expires
useUpstash: true,
globalCache: true,
userSpecific: false,
debug: true,
});
- User-Specific Cache with Expiration:
const userCacheMiddleware = createCacheMiddleware({
ttl: 3600, // 1 hour
useUpstash: true,
userSpecific: true,
debug: process.env.NODE_ENV === 'development',
});
This combination of simplicity and flexibility makes trpc-redis-cache adaptable to virtually any application scenario.
🎉 Why You Should Give it a Try
Caching can significantly improve your application’s performance, reduce latency, and enhance scalability. With trpc-redis-cache, you can do all this effortlessly, without sacrificing developer productivity or code quality. It’s designed with simplicity, clarity, and elegance at its core—enabling you to spend less time worrying about cache management and more time focusing on building great user experiences.
Feel free to explore, contribute, or share your feedback on GitHub!
👉 Explore the package on GitHub
Happy caching! 🚀